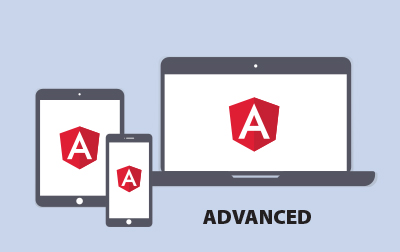
Advanced Angular Programming (ANG211)
This Advanced Angular Programming training class covers advanced topics of this next generation of the Angular framework. This course is intended for those that already have a basic understanding of the Angular 8 fundamentals and now want to cover more advanced features the Angular 8 framework offers.
Note: Although initially the 1st major revision of the AngularJS framework was known as "Angular 2" this is now technically incorrect as the Angular framework is releasing newer major versions (although the structure is still the same). Now "AngularJS" refers to the old architecture while simply "Angular" refers to the new architecture.
Manage Complexity: Do you have complex pages with many components and these components need to communicate with each other? Simple @Input and @Output mechanism is not enough for this. We will show you several strategies to manage this situation and come up with good solutions.
Slow Page Update: When you have many components on a page refreshing their templates can slow down updates. Learn how to optimize change detection which will definitely improve data updates.
Custom Validators: Do have custom input validation logic? How about doing real time validation of user input on the server side? This course delves deep into the validator API and shows you how to do these things.
Angular 8 Security: This is another popular topic that our customers keep asking us for. We show you the best practices for user authentication and authorization. We show how to prevent common attacks like XSS and CSRF.
- Advanced Component Concepts
- Detecting Change to @Input Binding
- Example Child and Parent Components
- Using a Custom Setter
- Using ngOnChanges
- Advanced Inter Component Communication
- Direct Access to Child
- Using a Template Local Variable
- Using the @ViewChild Decorator
- More About @ViewChild
- The @ViewChildren Decorator
- Live Monitoring of Children
- Direct Access to the Parent Component
- Communication Using Subject API
- Creating a Subject
- Publishing a Message in a Subject
- Subscribing to the Subject
- Problem With Ordering
- Content Projection
- Setup Projection Using ng-content
- Supplying Template for ng-content
- The Host Element
- Static Styling of the Host Element
- Setting DOM Properties of the Host
- Dynamically Loading a Component
- Dynamic Loading Example
- HostComponent Code
- Setting entryComponents
- Optimizing Change Detection
- Example Excessive Template Execution
- Using OnPush Change Detection Strategy
- Properly Changing @Input Variables
- Additional Notes on OnPush
- Summary
- Advanced RxJS
- Observable Creator Functions
- Subscribing to DOM Events
- The interval Function
- forkJoin and zip Function
- The concat Function
- The merge Function
- Recap of Operators
- The debounceTime Operator
- The distinct Operator
- The delay Operator
- The reduce Operator
- The flatMap Operator
- The switchMap Operator
- The retryWhen Operator
- Basic Example of retryWhen
- Retry for a Finite Time
- Creating a Custom Operator
- Advanced Example Custom Operator
- Intercepting All HTTP Calls
- Example Interceptor Service
- Registering the Interceptor
- Summary
- Custom Attribute Directive
- Recapping Attribute Directives
- Ways to Manipulate the Host Element
- Binding to the DOM Properties of the Host
- Listening for Host Events
- Obtaining the DOM Event Object
- Accessing the Host Component
- Adding @Input to a Directive
- Summary
- Custom Structural Directive
- How Do Structural Directives Work?
- Commonly Used API
- A Very Simple Conditional Directive
- Using the Sample Directive
- Passing Context Data to the Template
- Example Looping Directive
- Using the Looping Directive
- Summary
- Custom Validators
- Validator for Reactive Forms
- Example Validator Function
- Using the Validator
- Passing Data to Validator Function
- Validator for Template Driven Forms
- Example Validator Directive
- Using the Validator Directive
- Asynchronous Validator
- Example Asynchronous Validator Function
- Using the Validation Function
- Asynchronous Validator Directive
- Optimizing Asynchronous Validation
- Summary
- Distributing Angular 8 Libraries
- Introduction
- About Workspace and Project
- Creating a Library Project
- Anatomy of a Library Project
- Create a Component in the Library
- Create a Service in the Library
- Building the Library
- Distributing the Library
- Using the Library from an Application
- Using the Library Artifacts
- Writing a Sample Application
- Summary
- Internationalization (I18N)
- Introduction
- Translatable Text
- Extract Translatable Text
- Example XLIFF File
- Providing Translation
- Configure the Build System
- Serving and Building
- Deploying the Application
- Custom Translation Unit ID
- Getting the Current Locale
- Translatable Attribute Text
- Summary
- Security in Angular 8 Applications
- Overview of Authentication and Authorization
- About Identity Token
- Exchanging the Identity Token
- Example Login Code in Angular Service
- Options for Saving the Identity Token
- Sending the Token to Server
- Obtaining User Information
- Cross Site Scripting (XSS) Attack
- Angular and XSS
- Sanitizing Content
- Trusting HTML Content
- Cross-Site Request Forgery (XSRF)
- Preventing XSRF Using Angular
- Summary
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this Angular class:
- Some prior understanding of Angular fundamentals in addition to web development using HTML, CSS, and JavaScript. Experience developing with AngularJS (the prior version) is not required.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors