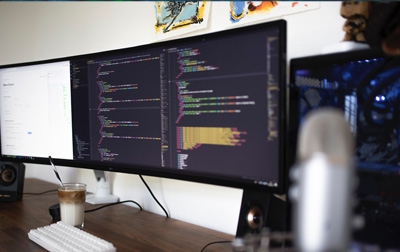
C++ Training (CPP101)
Course Length: 5 days
Delivery Methods:
Available as private class only
Course Overview
This C++ training class teaches students to design and write programs in the C++ language, emphasizing object-oriented approaches to designing solutions.
Course Benefits
- Learn to create Classes
- Learn to work with Constructors and Destructors
- Learn to understand Inheritance
- Learn about Virtual and Pure Virtual Functions
- Learn about References and Constants
- Learn about the new and delete keywords
- Learn to use Casting in C++
- Learn about Class Methods and Data
- Learn to create Overloaded Functions and use Overloaded Operators
- Learn about Exception Handling
- Learn to use the Standard Template Library
- Learn to work with STL Containers
Course Outline
- Classes
- Creating a Data Structure
- Methods
- Object Scope
- C++ Input and Output
- Namespaces
- Data Abstraction
- Enforcing Data Encapsulation
- File Organization
- Classes in C++
- Objects
- this Pointer
- Constructors and Destructors
- Debug Output
- The Default Constructor
- When are Constructors Called?
- The Destructor
- The Copy Constructor
- Other Constructors
- Why Did It Work Before?
- Composition
- The Report Class
- Code Reuse
- Initialization Lists
- Inheritance
- Inheritance
- Bugreport
- Protected Access Modifier
- Access and Inheritance
- Constructors and Inheritance
- Initialization Lists Revisited
- Multiple Inheritance
- Virtual Functions
- Inheritance and Assignment
- Inside Report's Assignment Operator
- Using Pointers - a Quick Look at Basics
- Class Assignment and Pointers
- Static Binding
- Dynamic Binding
- Polymorphism
- The show_rep() Function
- Using the show_rep() Function
- Designing Member Function Inheritance
- Pure Virtual Functions
- Bugfix and Its Relationship with Bugreport
- Bugfix: Association with Bugreport
- Using Bugfix with show_rep()
- Adding Bugfix to the Hierarchy
- Coding for the Document Class
- Reexamining the Document Class
- Pure Virtual Functions
- Updated: Designing Member Function Inheritance
- References and Constants
- References
- Displaying References
- Changing References
- Pass by Reference
- Returning by Reference
- Constant Variables
- Constant References
- Constant Methods
- new and delete
- new and delete
- Array Allocation
- The Report Class
- Compiler Version of the Copy Constructor
- Guidelines for Copy Constructors
- The Report Constructors and new
- The Report Destructor and delete
- Virtual Destructors
- Casting in C++
- Casting: A Review
- New Casting Syntax
- Creating a String Class
- The String Class
- The Conversion Constructor
- Expanding Our Casting Options
- Casting Operator
- Using the Casting Operator
- Class Methods and Data
- Class Data
- Class Methods
- Using the New Data
- More on Class Methods
- Overloaded Functions
- Function Overloading
- Using Overloaded Functions
- Rules for Overloading
- Overloading Based on Constness
- Default Arguments
- Invoking Functions with Default Arguments
- Overloaded Operators
- The Basics of Overloading
- Overloading operator+
- Coping with Commutativity
- Non-Commutative Operators
- friends and Their Problems
- The Assignment Operator
- Overloading the >> Operator
- Using Date with cout
- Exception Handling
- Why Exception Handling?
- try / catch / throw
- Exception Classes
- Standard Exception Hierarchy
- Multiple catch Blocks
- Catching Everything
- Unhandled Exceptions
- Exception in Constructors and Destructors
- Designing for Exceptions
- Standard Template Library
- Class Template Concepts
- Standard Template Library (STL) Overview
- Containers
- Iterators
- Iterator Syntax
- Non-Mutating Sequential Algorithms
- Mutating Sequential Algorithms
- Sorting Algorithms
- Numeric Algorithms
- auto_ptr Class
- string Class
- STL Containers
- Container Classes
- Container Class Algorithms
- vector Class
- Additional vector Class Methods
- deque Class
- list Class
- set and multiset Classes
- map and multimap Classes
Class Materials
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Class Prerequisites
Experience in the following is required for this C/C++ class:
- C or some other procedural language
Experience in the following would be useful for this C/C++ class:
- Java
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors