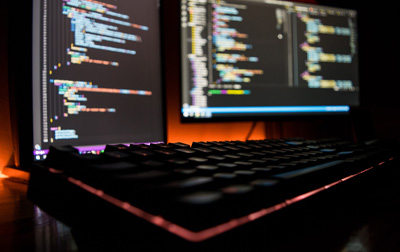
Developing Java EE Web Applications, plus JDBC/JPA and REST (JEE101)
This course provides a comprehensive guide to developing Java EE web applications with a focus on JavaServer Pages (JSP), Servlets, and data persistence using JDBC and JPA. It is designed for Java developers who want to build robust, scalable web applications that integrate seamlessly with databases, covering everything from the basics of web development to advanced topics like session management and transaction handling.
The course begins with an Introduction to Java EE, where you'll learn about the Java Enterprise Edition platform and its key components, including Servlets, JSP, and Enterprise JavaBeans (EJB). You'll explore the role of Java EE in enterprise application development and how it simplifies building large-scale, distributed applications.
In the Working with Servlets module, you'll dive into the fundamentals of Java Servlets, which handle requests and responses in a Java web application. You'll learn to create, configure, and deploy Servlets, manage request parameters, and handle HTTP methods like GET and POST. Practical exercises will guide you through building dynamic content, managing cookies, and understanding session tracking.
The JSP Development section focuses on JavaServer Pages, which allow you to create dynamic web content using HTML, CSS, and Java code. You'll learn how to use JSP tags, expressions, and directives to build interactive web pages. This module also covers JSP lifecycle, error handling, and integrating Servlets and JSPs for effective web application development.
The JDBC module teaches you how to connect Java applications to relational databases using Java Database Connectivity (JDBC). You'll explore the basics of JDBC API, including establishing connections, executing SQL queries, and managing result sets. You'll also learn best practices for handling exceptions and ensuring data integrity through transaction management.
In Advanced JDBC Features, you'll delve into topics such as using PreparedStatements for parameterized queries, CallableStatements for stored procedures, and batch processing for executing multiple queries efficiently. You'll also learn about connection pooling, which optimizes database connections for improved application performance.
The JPA and Object-Relational Mapping module introduces the Java Persistence API (JPA), which simplifies database interactions by mapping Java objects to database tables. You'll learn to define entities, manage persistence contexts, and use JPQL (Java Persistence Query Language) for querying data. This section emphasizes best practices for designing entities and managing relationships between them.
Transaction Management in Java EE explores how to ensure data consistency and integrity in web applications. You'll learn about local and global transactions, managing transaction boundaries, and how to implement transaction handling using JTA (Java Transaction API). This module also covers key concepts like isolation levels and concurrency control.
In the Security for Java EE Web Applications section, you'll learn about securing web applications using Java EE security features. This module covers authentication, authorization, role-based access control, and securing data with HTTPS. You'll also explore how to configure security constraints in web.xml and implement programmatic security checks.
The Web Application Deployment and Configuration module focuses on packaging and deploying Java EE web applications in various environments. You'll learn how to use web.xml for configuring Servlets, filters, and listeners, as well as deploying your application to popular Java EE servers like Apache Tomcat and WildFly.
The course concludes with Best Practices for Java EE Web Development, where you'll learn to design efficient, maintainable, and secure web applications. You'll explore patterns such as MVC (Model-View-Controller), DAO (Data Access Object), and Front Controller, and how to use these patterns to improve your application’s architecture.
By the end of this course, you'll have the skills to develop fully-functional Java EE web applications that connect to databases using JDBC and JPA. You’ll be able to build scalable, secure, and efficient applications that meet enterprise standards, preparing you to tackle complex web development challenges with confidence.
- Design and build robust and maintainable web applications
- Create dynamic HTML content with Servlets and Java ServerPages, using the JSP Standard Tag Library (JSTL)
- Make Servlets and JSP work together cleanly
- Use JSTL and other Custom Tag Libraries to separate Java and HTML code
- Access databases with JDBC and the JPA (Java Persistence API)
- Structure a clean data access layer.
- Gain a high level understanding of REST services and JAX-RS
- Java EE Introduction
- Java EE Overview and Technologies
- JEE Architecture Choices
- Java Web App Introduction
- Servlet Basics
- Servlet Basics and Capabilities
- Basics of Writing a Servlet
- HTML Forms Review
- HTTP Review: Request-response, headers, GET, POST
- Overview: How Servlets Work
- Servlet Lifecycle and API - Servlet, HttpServlet, @Webservlet
- Requests and Responses - HttpServletRequest and HttpServletResponse
- Accessing Parameters
- web.xml
- Additional Servlet Capabilities
- Working with HttpServletResponse (Errors, Headers, MIME types)
- Initialization
- Overview
- Using ServletConfig and ServletContext
- Init Parameters - Servlet and Web App
- Error Handling: Error Pages and Their Configuration
- JavaServer Pages
- Basics and Overview
- JSP architecture
- JSP tags and JSP expressions
- Fixed Template Data
- Lifecycle of a JSP
- Model View Controller (MVC)
- Overview
- Java EE Model 2 Architecture
- Servlets as Controllers, RequestDispatcher, Forwarding and Including
- Data Sharing in a Web App
- Object scopes or "buckets"
- Using JavaBeans to Hold Data
- Using the Scope Objects - get/set/remove Attributes
- Request, application, session and page scope
- JSP Expression Language (EL) and Data Access
- JSP EL Overview
- JSP Expressions, and Accessing Data
- Predefined JSP EL implicit objects (pageContext, param, header, scope objects)
- pageContext in Detail
- jsp:include, jsp:forward, the page Directive
- JSP Error Pages
- Using Custom Tags
- Custom tags overview
- Tag Libraries Overview
- taglib Directive - Using a Tag Library
- JSTL
- Overview
- c:out, c:forEach
- c:url, c:param
- More about JSTL
- Other Useful Core Tags < - c:if, c:choose
- Formatting: formatNumber, formatDate, Resource Bundles
- HTTP Session Tracking
- HTTP and Client State
- Cookies - Overview, Servlet Access, Usage, Issues
- Sessions
- Servlet/JSP Session Support, HttpSession
- Using Sessions - Putting Data in, Retrieving Data From
- How Sessions Work
- Web Security
- JEE Security Overview
- Role Based Security
- Declarative Security
- Web Authentication - Basic, Form-Based, Digest, HTTPS Client
- Using Basic Authentication
- Using Form-Based Authentication
- Programmatic Security - HttpServletRequest, Retrieving Roles
- Additional Servlet Capabilities
- Custom Tags Using Tag Files - Overview, Writing and Using Tag Files, Tag Attributes
- Servlet Filter overview - Example and lifecycle
- WebSocket Overview
- JDBC Introduction and Architectur
- Relational Database and JDBC Overview
- JDBC Architecture, JDBC API Overview
- Connecting to a database
- Working with JDBC - Executing Statements and Processing Results
- Resource Integration
- DataSources and Connection/Statement Pooling
- Resource Injection, @Resource, and JNDI
- CDI and Dependency Injection
- Introduction to JPA
- JPA Architecture and Programming View
- Entity Classes and Annotations
- Mapping an Entity Class
- EntityManagerFactory and EntityManager
- Working with JPA (Find by primary key and inserts)
- Integrating JPA with the Web Tier
- JPA Advanced Topics
- Entity Lifecycle
- Relationships
- Issues with Relationships (Lazy Load and Web Apps)
- Introduction to REST
- Overview and Principles
- REST Characteristics
- Resources and Operations
- REST Principles
- Requests and Responses
- REST APIs
- URI Templates
- GET, POST, PUT, DELETE
- Safe and Idempotent Methods
- Introduction to JAX-RS
- APIs and Implementations
- JAX-RS Overview, Annotations
- JAX-RS Implementations
- Runtime Environment
- Application Server, Servlet-Only Container
- Architectural and Implementation Perspectives
- Configuring the Application
- Applications, Resources, and Providers
- JAX-RS Applications
- Resource Classes and @Path
- Provider Classes and @Provider
- Default Lifecycles
- The Application Class and rest-path
- Ajax-JavaScript Clients
- Overview
- Classic vs. Ajax Interactions
- Working with Ajax-JavaScript
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors