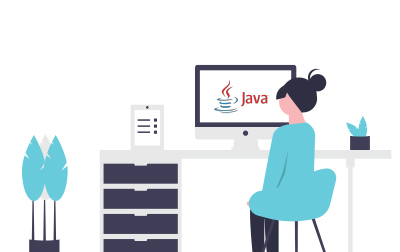
Intermediate/Advanced Java Training (JVA104)
This modern, fast-paced Intermediate Java Programming course is suitable for developers with previous experience in Java and Object-Oriented (OO) programming. It is also ideal for developers proficient in other OO languages, such as Python, C++, or C#, who want to deepen their Java skills. The course includes numerous optional parts, allowing customization based on your group’s needs, making it flexible enough for advanced Java developers seeking to enhance their knowledge.
The course begins with an accelerated yet thorough hands-on review of Java foundational concepts, focusing on OO design and implementation principles. This review covers essential topics such as Java syntax, control structures, and object-oriented principles, alongside newer language features like the Date/Time API (Java 8+), type inference with var, and switch expressions. This section ensures all participants are aligned on core Java concepts before moving into more complex material.
Next, you’ll explore Advanced Object-Oriented Programming (OOP) concepts, including inheritance, polymorphism, abstract classes, interfaces, and design patterns such as Singleton and Factory. You’ll learn to make critical decisions about using composition versus inheritance, a key skill for creating well-structured OO systems. This section emphasizes OO design principles that enhance code maintainability and scalability.
The Java Collections and Generics module covers advanced data structures like linked lists, hash maps, and trees, with a focus on type-safe collections using generics. You’ll learn to implement custom collections, optimize data storage, and perform complex data manipulations, enhancing your ability to work efficiently with data.
In the Functional Programming module, you’ll dive into lambdas, streams, and functional interfaces, learning to write cleaner, more efficient code. You’ll also explore the Java Platform Module System (JPMS), which represents a fundamental shift in application organization and library integration. Migration strategies and a step-by-step case study provide practical insights into adopting JPMS in existing projects.
Exception Handling and Debugging are emphasized throughout, where you’ll learn advanced techniques for managing exceptions and using try-with-resources for I/O operations. You’ll gain hands-on experience in debugging with IDE tools, step through code, and optimize performance, essential skills for professional Java development.
The Input/Output (I/O) and Serialization module teaches you to read and write files, manage streams, and handle object serialization, ensuring you can manage data efficiently within your applications. The Networking and Sockets section covers client-server application development, focusing on TCP and UDP protocols, sockets, and secure data exchange.
Concurrency and Multithreading are explored in-depth, focusing on creating responsive applications that perform multiple tasks simultaneously. You’ll learn about thread synchronization, the Executor framework, and techniques for avoiding concurrency pitfalls like deadlock. This module prepares you to build high-performance applications that leverage Java’s concurrency capabilities.
The Database Integration with JDBC module covers connecting Java applications to relational databases, performing CRUD operations, and managing database connections. This section equips you with the skills to integrate Java with databases, ensuring data is handled efficiently and securely in your applications.
JavaFX and GUI Programming provide an introduction to building interactive graphical user interfaces, with coverage of scene graphs, layout managers, and event handling. This module helps you develop visually appealing and user-friendly applications that enhance the overall user experience.
Beyond core Java programming, the course includes important topics such as UML and Design Patterns, equipping you with the skills to model and design complex systems effectively. You’ll also learn about best practices in Java development, including code optimization, test-driven development (TDD) with JUnit, and clean code principles.
Unit testing is integrated throughout the course, with most labs implemented as JUnit tests, reinforcing the importance of testing in software development. The hands-on nature of the course, with numerous code examples and programming labs, ensures you can immediately apply what you’ve learned to your current projects.
This course is designed to be highly flexible and can be tailored to fit your specific learning needs. Be prepared to work hard, dive deep into advanced Java topics, and significantly enhance your programming skills, setting you on the path to becoming a more proficient and productive Java developer.
- Solidify Java foundational knowledge, including the important contracts of class Object.
- Understand the uses and consequences of inheritance and composition, and reinforce the role of interfaces.
- Reinforce fundamental OO principles such as cohesion, coupling, and polymorphism.
- Use the JUnit testing framework and become fluent in writing assertions to verify correct program behavior.
- Familiarity with UML modeling in class diagrams and sequence diagrams.
- Use advanced techniques for object creation, including factories and singletons.
- Use established design patterns for object composition, including Strategy, Decorator, and Facade.
- Write and use generic classes and methods.
- Learn the use cases for inner classes and refactor existing code to use them when appropriate.
- Create and use custom annotations.
- Be familiar with reflection and how to use it.
- Understand the role of functional interfaces.
- Understand lambda expressions and method references, and use them to pass behavior (methods).
- Use the Stream API to perform complex processing of collections and other input sources.
- Create and use Java modules, understanding module descriptors, modular JARs, exports and dependencies, and the modulepath.
- Understand the structure and behavior of the modular JDK, and how it supports modular applications as well as legacy classpath-based code.
- Migrate classpath-based applications to Java 11, understanding the stages of migration and options available.
- Java State of the Union
- Java Release Cycle
- New Java Versions
- Review - Basics
- Java Environment
- Classes and Objects
- Instance Variables, Methods, Constructors, Static Members
- OO Principles: Data Encapsulation, Cohesion
- Object Contracts: toString(), equals() and hashCode(), Comparable and Comparator
- Packages, Enums, Arrays
- Exceptions
- Date and Time API
- New Language Features
- Review (Inheritance and Interfaces)
- UML Overview
- Inheritance
- Definition and IS-A Relationship
- Method Overriding, @Override
- OO Principles: Principle of Substitutability, Polymorphism and Encapsulation of Type, Coupling, Open-Closed Principle
- Constructor Chaining
- Interfaces
- Defining and Implementing, Interface Types
- Interface Inheritance
- New Interface Features (Java 8+)
- Default Methods, Static Methods
- Functional Interfaces
- Guidelines
- JUnit
- Overview
- Tests, Assertions, and Fixtures
- Writing and Running Tests
- Assertions
- Test Fixtures, @Before and @After, @BeforeClass and @AfterClass
- Testing for Exceptions
- Best Practices and Test-Driven Development Overview (TDD)
- Collections and Generics
- Collections Overview
- Generics and Type-Safe Collections
- Diamond Operator
- Lists, Sets, and Maps
- Interfaces and Contracts
- Iteration and Autoboxing
- Utility Classes - Collections and Arrays
- Writing Generic Classes
- Inheritance with Generic Types
- Wildcard Parameter Types
- Type Erasure
- Collections Overview
- Techniques of Object Creation
- Design Patterns Overview
- Controlling Object Creation
- Limitations of new Operator, Alternative Techniques
- Singleton Pattern
- Simple Factory
- Factory Method Pattern
- Other Techniques
- Named Objects, JNDI
- Dependency Injection Frameworks
- Using Composition and Inheritance Effectively
- Inheritance and Composition - Pros and Cons
- Composition and Delegation
- HAS-A, USES Relationships
- Strategy Pattern
- Decorator Pattern
- Façade and Other Patterns
- Façade, Proxy, Template Method
- Inheritance and Composition - Pros and Cons
- Inner Classes
- Overview and Motivation
- Stronger Encapsulation, Rules and Caveats
- Defining and Using Inner Classes
- Member-Level, Method-Local, Anonymous Classes
- Static Nested Classes
- Nested Classes, Nested Interfaces, Nested Enums
- Overview and Motivation
- Annotations
- Overview
- Using Annotations
- Target and Retention Policy
- Annotation Parameters, Parameter Shortcuts
- Writing Custom Annotations
- Syntax, Using the Meta-Annotations
- Using a Custom Annotation
- Reflection
- Overview and API
- The Class Called Class
- Obtaining and Inspecting Class Objects
- Working with Objects Reflectively
- Creating Instances, Invoking Methods, Setting Field Values
- Overview and API
- Lambda Expressions
- Functional Interfaces and Lambdas
- Target Context
- Using Lambda Expressions
- Syntax, Lambda Compatibility
- Variable Capture
- Type Inference
- Method References
- Three Types of Method References
- Refactoring Lambdas into Method References
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this Java class:
- Working knowledge of Java programming, including use of inheritance, interfaces, and exceptions
Courses that can help you meet these prerequisites:
Private Class Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors