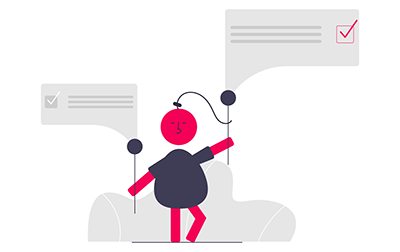
New Features in Java (JVA501)
This course provides an in-depth exploration of Java's modular system and the new features introduced in recent Java versions. It is tailored for experienced Java developers who want to understand and implement Java Modules, leverage type inference, and utilize new tools and APIs like JShell and the updated HttpClient. The course covers practical techniques and best practices, helping you stay current with modern Java development standards.
The course begins with an Introduction to Modules, where you’ll learn the motivation behind the Java Platform Module System (JPMS). You'll explore different types of modules, the modular JDK, and how modularization helps improve application performance and maintainability. This section sets the stage by explaining the benefits of adopting modular architecture in your projects.
In the Working with Java Modules section, you'll gain hands-on experience in defining and using Java modules. You’ll learn how to create module definitions, manage dependencies, and use services to build loosely coupled applications. The module also covers compatibility and migration strategies for transitioning legacy applications to a modular structure, ensuring smooth integration of modularity into your existing codebase.
The Type Inference module introduces local-variable type inference with the var
keyword, a feature that simplifies Java syntax without sacrificing type safety. You’ll explore how type inference works, its limitations, and its impact on readability and maintainability. The section also provides a brief overview of lambdas and introduces local-variable syntax for lambda expressions, enhancing your ability to write concise and expressive code.
The JShell section covers the Java Shell tool, an interactive REPL (Read-Eval-Print Loop) introduced in Java 9. You'll learn how to use JShell to experiment with code, test snippets, and explore new APIs in an interactive environment. The module also explains how to use external libraries, including modules and JAR files, within JShell, making it a valuable tool for rapid prototyping and testing.
In the HttpClient module, you’ll explore the updated HttpClient API, which provides a modern, efficient way to handle HTTP requests in Java. You'll learn about the key features of the new API, including asynchronous requests, HTTP/2 support, and simplified usage patterns. Practical exercises will guide you through building applications that communicate with web services using this powerful API.
The Other New Features section highlights additional updates in recent Java versions, including collection factory methods, multi-release JARs, and reactive programming enhancements. You’ll learn how these features simplify common programming tasks, improve code flexibility, and allow for more responsive application designs. This module also covers miscellaneous updates that enhance Java’s overall functionality and developer experience.
Finally, the Custom Runtime Images module teaches you how to create application-specific runtimes using jlink
. You'll learn the benefits of custom runtime images, such as reduced application size and improved security. The section guides you through the process of building a custom runtime tailored to your application's needs, enabling you to optimize performance and resource usage.
By the end of this course, you’ll have a thorough understanding of Java’s modular system and the latest features in the Java platform. You’ll be equipped to write cleaner, more efficient code, leverage new tools and APIs, and modernize your applications to take full advantage of the latest Java advancements.
- Understand the new Java release cycle and Long Term Support (LTS) releases.
- Be familiar with significant deprecated and removed features, and how to work around them.
- Create and use Java modules, understanding module descriptors, modular JARs, exports and dependencies, and the
modulepath
. - Understand the structure and behavior of the modular JDK, how it supports modular applications as well as legacy
classpath
-based code, and the implications of strong encapsulation on each. - Migrate
classpath
-based applications to Java 11/17, understanding the stages of migration and options available. - Recognize the issues with 3rd party libraries in a migration effort, and how to work with them on the modulepath and
classpath
. - Use local-variable type inference with
var
, includinglambda
parameters. - Gain a practical working knowledge of the JShell REPL tool, including working with code snippets and variables, configuration, and using external libraries.
- Use the HTTP Client to access HTTP resources from Java, as well as understand the other options available.
- Work with various HTTP request and response types, using both synchronous and asynchronous techniques.
- Outline the new factory methods in Java Collections and understand native immutable collections.
- Describe the motivation for multi-release JAR files (MR-JARs), understand their structure, and how to create them.
- Understand the runtime behavior of MR-JARs in both legacy and modern JVMs, and principles and strategies for working with them effectively.
- Outline the principles of Reactive Programming and how it differs from traditional synchronous invocation models.
- Describe Reactive Streams and the role of the Flow API that defines the Java platform’s support for them.
- Understand the characteristics and benefits of custom runtime images.
- Use
jdeps
to analyze application dependencies, and create custom runtimes withjlink
, for both modular andclasspath
-based applications. - Explore some of the more important additional features and APIs, including new features for interfaces, the Process API, new JDK tools and command line options.
- Introduction to Modules
- Motivation and Overview
- Types of Modules
- Modular JDK
- Our Approach
- Working with Java Modules
- Defining and Using Modules
- Services
- Compatibility and Migration
- Conclusion
- Type Inference
- Local-Variable Type Inference
- Brief Overview of Lambdas
- Local-Variable Syntax for Lambdas
- JShell
- Introduction to JShell
- Working with Code
- Using Libraries (Modules, Jars, etc.)
- Http Client
- Overview
- API
- Usage and Features
- Other New Features
- Collection Factory Methods
- Multi-Release JARs
- Reactive Programming
- Miscellaneous
- Custom Runtime Images
- Application-Specific Runtimes
- Benefits
- Creating Runtime Images with
jlink
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this Java class:
- Working knowledge of Java programming, including use of inheritance, interfaces, and exceptions.
Courses that can help you meet these prerequisites:
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors