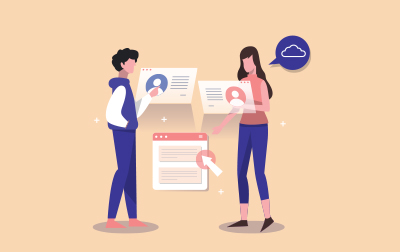
Creating, Styling, and Validating Web Forms (WFM101)
This course offers a comprehensive guide to working with HTML forms, from creating and styling forms to validating user input using JavaScript and server-side technologies. It is ideal for web developers who want to gain a deep understanding of form handling and validation in both client-side and server-side contexts.
The course begins with an introduction to HTML Forms, covering the basics of how forms work, the form element, and various form elements like buttons, checkboxes, radio buttons, select menus, and textareas. You'll learn how to create a registration form and enhance your forms with fieldsets for better organization. Practical exercises will help you apply these concepts, and you'll also explore how to style forms with CSS to improve their appearance.
In the JavaScript Form Validation module, you'll learn the difference between server-side and client-side form validation. The focus will be on using JavaScript to validate form data, including checking the validity of email and URL fields, and handling validation on input and submit events. You'll also learn how to add error messages and validate various form elements like textareas, checkboxes, radio buttons, and select menus. Practical exercises, such as validating an ice cream order form, will give you hands-on experience.
The Styling Forms with CSS section dives into techniques for enhancing the look and feel of your forms. You'll learn about general form layout, applying pseudo-classes to form fields, and styling specific elements like radio buttons, checkboxes, and fieldsets. Exercises will help you put these styling techniques into practice.
The Regular Expressions module introduces you to the powerful tool of regular expressions, which are used for pattern matching within strings. You'll learn the syntax of regular expressions, how to use backreferences, and how to apply regular expressions to form validation. This section also covers cleaning up form entries and includes exercises to help you master these techniques.
Next, you'll explore Node.js and Server-side Form Validation. This section introduces server-side programming, starting with the basics of Node.js and setting up a simple web application. You'll learn about processing forms on the server, performing validation using Node.js and the Express framework, and handling Ajax requests for asynchronous form validation. Exercises will guide you through creating and validating forms on the server side.
The course concludes with a module on JSON, where you'll learn about JavaScript Object Notation (JSON), a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. You'll review object literals and work with JSON in the context of form data, with exercises to reinforce your understanding.
By the end of this course, you'll have the skills to create, style, and validate forms using both client-side and server-side techniques, ensuring that your web applications handle user input securely and effectively. You'll also gain a solid understanding of JSON, enabling you to work with data in a structured and efficient way.
- Learn to create HTML forms using the latest HTML standards.
- Learn to style forms with CSS.
- Learn to validate forms on the client with JavaScript and regular expressions.
- Learn to validate forms on the server with Node.js.
- Learn to use Ajax techniques to do server-side validation on specific form fields without refreshing the entire page.
Private classes are delivered for groups at your offices or a location of your choice.
Learn at your own pace with 24/7 access to an On-Demand course.
- HTML Forms
- How HTML Forms Work
- The <form> Tag
- Get vs. Post
- Form Elements
- id and name Attributes
- Text Fields
- Labels
- Text-like Input Types
- placeholder Attribute
- pattern Attribute
- Password Fields
- Date and Time Fields
- Number Fields
- Color Fields
- Tel, URL, and Email Fields
- tel
- url and email
- Search Fields
- Hidden Fields
- Buttons
- Submit Button
- Reset Button
- Button Buttons
- Checkboxes
- Radio Buttons
- Fieldsets
- Select Menus
- Option Groups
- Textareas
- JavaScript Form Validation
- Server-side Form Validation
- HTML Form Validation
- Accessing Form Data
- Form Validation with JavaScript
- Checking Validity on Input and Submit Events
- Adding Error Messages
- The dataset Property
- Validating Textareas
- Validating Checkboxes
- Validating Radio Buttons
- Which Radio Button was Selected?
- Validating Select Menus
- Giving the User a Chance
- Styling Forms with CSS
- General Form Layout
- Form-field Pseudo-Classes
- Applying Pseudo-Classes to Forms
- Radio Buttons, Checkboxes, and Fieldsets
- Regular Expressions
- Getting Started
- JavaScript's Regular Expression test() Method
- Regular Expression Syntax
- Start and End ( ^ $ )
- Number of Occurrences ( ? + * {} )
- Common Characters ( . \d \D \w \W \s \S )
- Grouping ( [] )
- Negation ( ^ )
- Subpatterns ( () )
- Alternatives ( | )
- Escape Character ( \ )
- Case-Insensitive Matches
- Backreferences
- Form Validation with Regular Expressions
- Cleaning Up Form Entries
- A Slightly More Complex Example
- Getting Started
- Node.js and Server-side Form Validation
- Welcome to the Server-side
- What is a web server?
- Dynamic Websites
- Google Chrome DevTools: Network Tab
- Status Codes
- Welcome to Node.js
- Installing Node.js
- package.json
- Our First App
- What does npm start do?
- Our First Web App
- Stopping the Server
- Fat-arrow Functions
- Sending a Response with HTML
- The favicon.ico Icon
- Simple Routing and 404 Pages
- Delivering favicon.ico
- Express - Node.js Web Application Framework
- app.js
- Favicon Middleware
- Static Files
- Processing a Simple Form
- Form Validation
- Validators
- Validation Chaining
- not()
- withMessage(message)
- Custom Validators
- Ajax
- XMLHttpRequest
- Asynchronous
- Welcome to the Server-side
- JSON
- Review of Object Literals
- Arrays
- Objects
- Arrays in Objects
- Objects in Arrays
- Back to JSON
- JSON Syntax
- The built-in JavaScript JSON Object
- Review of Object Literals
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this JavaScript class:
- Basic HTML
- Basic CSS
- Basic JavaScript
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors
Self-Paced Course
- On Demand 24/7
- Readings
- Presentations
- Exercises
- Quizzes
- Full Year of Access
- Learn more