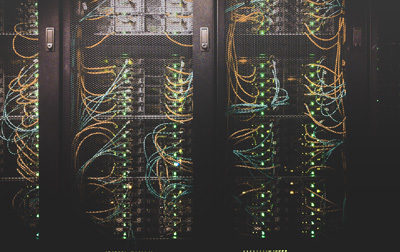
Introduction to React and Redux (REA112)
Course Length: 3 days
Delivery Methods:
Available as private class only
Course Overview
This 3-day Introduction to React training class gets students up to speed quickly with React.
Course Benefits
Course Outline
- Setup
- Verifying Node.js and either NPM or yarn
- Verifying class libraries
- Verifying class files
- IDE (WebStorm or Visual Studio Code preferred)
- Introduction to React
- What problem(s) does React solve?
- Traditional, pre-JS web applications
- Late-model, MV* and JS web applications
- React's solutions
- Single-page apps
- View libraries
- Helper libraries
- React development environment
- Simplicity: create-react-app
- Build-your-own: an overview
- Hello world
- Your first React component
- Using React within a page
- Making some basic changes
- React and JSX
- What problem(s) does React solve?
- Components
- Two types of components
- Functional components
- Class-based components
- Why use one or the other?
- Testing basic components
- Testing libraries: Enzyme vs Testing Library (sic)
- Jest
- Testing with Testing Library
- Props and state
- Passing in properties
- Limitations of properties
- Using state and the useState() hook
- When to use state, when to use props
- Testing state and prop changes
- Event handling
- React event handling
- Synthetic events
- React vs DOM event handling
- Testing events
- Children
- Components within components
- Known children and unknown children
- Testing child components
- Parent-child component communication
- Communication from parent to child
- Communication from child to parent
- Container vs presentational components
- Two types of components
- React Component Lifecycle
- Lifecycle overview
- Startup and mounting
- Rendering
- Updating
- Unmounting
- Using useEffect() for lifecycle methods
- Run once
- Run every render
- Run on specific changes / updates
- Lifecycle methods in tests
- Error handling and error boundaries
- Lifecycle overview
- Intermediate component usage
- PropTypes
- Typing and React
- Using PropTypes
- PropTypes in production
- PropTypes vs TypeScript
- Asynchronous data
- When should asynchronous fetching be done?
- What challenges does async offer?
- Asynchronous best practices
- Testing against async fetches
- Lists of data
- Iterating over a list
- The key property
- Sorting data
- Testing component interactions
- PropTypes
- Forms
- Controlled vs uncontrolled components
- What does React know about your form field?
- Does React control your form field?
- When does React find out about changes to your form field?
- Form field types
- Controlling a text field
- ther form fields
- Getting data out of a form
- Working with form data in tests
- Controlled vs uncontrolled components
- Introduction to Redux
- What problems does Redux solve?
- How does it solve them?
- Basic Redux pattern
- Store
- Reducers
- Actions
- A basic Redux example
- What problems does Redux solve?
- Modern Redux with the Redux Toolkit
- What is the Redux toolkit
- What does it provide?
- The ducks pattern
- Testing Redux
- React and Redux
- Plugging into React
- State as props
- Events as dispatch
- Introducing higher-order components
- Turning our standalone Redux program into a component
- Middleware
- Provided by the toolkit
- ther middleware
- Building a real-world React-Redux component
- Testing React-Redux components
- Higher-order components in detail
- What do higher-order components do?
- Why would I use a higher-order component?
- Plugging into React
- Asynchronous Redux
- The difficulties of asynchronous Redux
- Asynchronous middleware
- Depending on your needs, we can use either thunks, sagas, or survey both techniques for asynchronous interactions
- Dispatching async actions
- Catching results
- Handling errors
- Testing asynchronous Redux
Class Materials
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Class Prerequisites
Experience in the following is required for this JavaScript class:
- 1-2 years of JavaScript experience.
- Advanced understanding of JavaScript, including prototypes and functions as first class citizens.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors