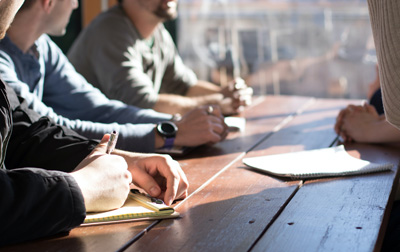
Objective-C Training for iOS Development (OBJC101)
Course Length: 4 days
Delivery Methods:
Available as private class only
Course Overview
This Objective-C training class teaches the fundamentals of programming in Objective-C, the language used to develop iPhone and iPad applications. This course covers Xcode, the integrated development environment used to build Objective-C applications.
Course Benefits
- Learn how to perform unit testing.
- Learn to work with flow control.
- Learn how to perform debugging.
- Learn to work with windows and views.
- Learn to work with collections.
- Learn to manipulate design and work with picker view and date picker.
- Learn to work with directories and files.
- Learn to work with MapKit and Networking.
- Learn to run you app on a physical device and deploy.
Course Outline
- Getting Started
- Introduction to Objective-C
- Lab: Hello World
- Data Types and Variables
- Lab: Properties
- Constants
- Alloc-Init & New
- Strings, Numbers, Bool
- Literals
- Typecasting
- nil
- Unit Testing
- Introduction
- XCTest
- Performance Tests
- Lab: Unit Tests
- Xcode Server
- Create Bot
- Lab: Xcode Server
- More Objective-C: Flow Control
- Loops
- Switch
- Lab: Loops
- Logical Operators
- UI with Interface Builder
- Introduction
- View Controllers
- View
- Connections
- Lab: Connections
- More Objective-C: Functions
- Functions
- Parameters
- Lab: Functions
- Blocks
- Completion Handlers
- Lab: Blocks
- Debugging
- Introduction
- Breakpoints
- Debug Gauges
- Debug Area/Console
- Lab: Debugging
- Classes
- ARC
- Initializers
- Windows and Views
- Windows
- Views
- Responder Chain
- View Resizing
- Screen Size Considerations
- Auto Layout
- Lab: Constraints
- More Objective-C: Collections
- Arrays
- Sets
- Dictionaries
- Enumerations
- Lab: Collections
- Asset Management
- Asset Catalogs
- App Icon
- More Objective-C: Beyond Basics
- Protocols
- Lab: Protocols
- Categories
- Application Patterns
- Model-View-Controller
- Model-View-Presenter
- Model-View-ViewModel
- Target-Action
- Subclassing
- Delegation
- Protocol Oriented Programming
- Storyboards
- Introduction
- Segues
- Passing Data
- Lab: Storyboards
- UI Design
- Constraints
- Table Views
- Table View Styles
- Cell Styles
- Prototype Cells
- Table Views
- Navigation
- Static Table Views
- Lab: Table View
- Universal Apps
- Picker View
- Date Picker
- Directories and Files
- Introduction
- Paths
- Paths and Directories
- File I/O
- User Defaults
- Lab: Files
- CoreData
- CoreData
- Entities
- CoreData
- Core Generation
- Lab: CoreData
- Touches, Taps, and Gestures
- Touches
- Gestures
- Lab: Touches
- Animation
- Lab: Animations
- App States
- App States
- App Delegate
- Considerations/Limitations
- Background Execution
- Notifications
- Overview
- Permission
- Local Notifications
- Push Notification
- Notification Center
- CoreLocation
- Lab: CoreLocation
- MapKit
- MapKit
- Concurrency
- Operations and Operation Queue
- NSInvocationOperation
- NSBlockOperation
- Grand Central Dispatch
- Lab: Concurrrency
- Networking
- Reachability
- Asynchronous Downloads
- Lab: Downloads
- GET Request
- POST Request
- JSON Data
- Lab: JSON
- Localization
- Resources
- Translation Considerations
- Language and Region
- Running on a Physical Device
- Developer Account
- Device
- Performance and Power Optimization
- Performance
- Measuring Performance
- Memory Considerations
- Network Considerations
- Deployment
- Icons and Launch Storyboard
- Archiving
- Distributing
- iTunesConnect
- User Feedback
- Obj-C from Swift
- Swift from Obj-C
Class Materials
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Class Prerequisites
Experience in the following is required for this Objective-C class:
- Basic computer experience.
Experience in the following would be useful for this Objective-C class:
- Some experience in a programming language.
- Broader exposure than HTML, CSS, etc. is strongly suggested.
- If unsure about your experience and prerequisites, contact Webucator customer service who can put you in touch with an Objective-C instructor.
- End-user experience with an iPod, iPhone, or iPad.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors