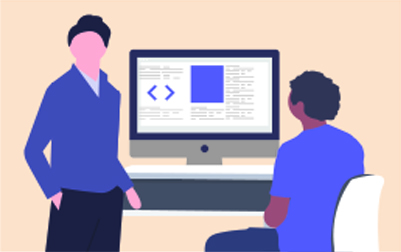
Introduction to PHP Training (PHP101)
This PHP course is designed to give you a comprehensive understanding of PHP, a powerful server-side scripting language widely used to create dynamic and interactive websites. Whether you’re new to programming or you’re looking to strengthen your PHP skills, this course will take you from the basics to more advanced concepts, providing practical knowledge you can apply directly to your work environment.
We begin with PHP Basics, which introduces you to the server-side of web development, starting with how web servers function and their role in delivering dynamic websites. You'll get acquainted with Google Chrome DevTools, specifically the Network tab for understanding status codes, and learn the ins and outs of how PHP operates, including configuring the php.ini file and writing your first PHP script. Topics such as PHP statements, whitespace, functions, and the use of php.net for documentation are covered in a way that's accessible to beginners.
The next module dives into Variables, where you'll explore variable types, naming conventions, and the concept of type juggling and type casting. You'll understand the differences between single and double quotes, how to concatenate strings, and how to pass variables through the URL. Later, you'll build on this knowledge with user-defined functions, learning to define and call functions, set default values, and distinguish between passing by reference and passing by value.
In Including Files, you’ll learn how to use require
and require_once
directives to manage code more efficiently by including external files. You'll also discover how to use constants and handle errors through error reporting and displaying error messages, ensuring your code is robust and less prone to failures.
The course continues with PHP Conditionals, essential for controlling the flow of your PHP programs. You’ll cover various conditional statements, such as if, if-else, and switch-case, and understand falsy values and testing for variable existence. Moreover, the course introduces the ternary and null coalescing operators to simplify your conditional expressions.
Next, you'll study Arithmetic Operators and Loops. This lesson covers arithmetic and modulus operators and introduces different looping constructs, including while, do...while, and for loops, as well as how to effectively use break and continue statements.
The module on Arrays goes into detail about different types of arrays, such as indexed, associative, and multi-dimensional arrays. You’ll learn how to initialize, append, read, and loop through these arrays, in addition to using built-in array manipulation functions.
In Working with Databases, you'll be guided through the concepts of objects, attributes, behaviors, and classes, and learn how to connect to a database using PDO. You'll practice querying records, adding pagination, and implementing sorting and filtering right within your PHP scripts, using a sample Poetree database for hands-on experience.
The course also includes a comprehensive look at Exception Handling. You'll learn how to manage uncaught exceptions, throw and catch custom exceptions, and handle errors specifically related to database operations with PDOExceptions.
PHP and HTML Forms covers how to handle HTML form submissions securely, by sanitizing and validating form data. You’ll explore methods such as htmlspecialchars()
, htmlentities()
, and filter_var()
, ensuring user input is safe and reliable.
The lesson on Sending Email with PHP introduces you to the built-in mail() function and the more advanced PHPMailer library. You’ll set up a mail server and use PHPMailer to send emails, including creating a contact form and customizing email methods and properties.
Next, the module on Authentication with PHP and SQL teaches you how to create a secure registration and login system using sessions and cookies. You’ll also cover password management, implementing form-based authentication, and handling session variables.
The hands-on Lab focuses on inserting, updating, and deleting poems in the Poetree database, giving you real-world experience with CRUD operations within a PHP environment.
The final lesson on Uploading Files shows you how to handle file uploads through HTML forms, including resizing images and uploading profile pictures, to complete your PHP toolkit.
By the end of this course, you’ll have a firm grasp of PHP fundamentals and the ability to build dynamic, server-side web applications. You’ll be equipped with practical skills to make your web projects more interactive and responsive, laying a strong foundation for further PHP or full-stack development endeavors.
- Learn how PHP works.
- Learn the basic syntax of PHP.
- Learn to create dynamic interactive pages with PHP.
- Learn to handle exceptions in PHP.
- Learn to work with arrays in PHP.
- Learn to process and validate forms with PHP.
- Learn to write functions in PHP.
- Learn to manipulate and manage database data with PHP.
- Learn to manage sessions and cookies with PHP.
- Learn to create a complete and secure registration process with PHP.
- Learn to send email with PHPMailer.
- Learn to upload files with PHP.
Private classes are delivered for groups at your offices or a location of your choice.
Learn at your own pace with 24/7 access to an On-Demand course.
- PHP Basics
- Welcome to the Server-side
- What is a web server?
- Dynamic Websites
- Google Chrome DevTools: Network Tab
- Status Codes
- How PHP Works
- The php.ini File
- PHP Tags
- Hello, World!
- Comments
- PHP Statements and Whitespace
- PHP Functions
- php.net
- Variables
- Variable Types
- Variable Names (Identifiers)
- Type Juggling and Casting
- Hello Variables!
- Variable Scope
- Superglobals
- Single Quotes vs. Double Quotes
- Concatenation
- Passing Variables on the URL
- User-defined Functions (UDFs)
- Defining and Calling Functions
- Default Values
- Variable Scope
- By Reference vs. By Value
- Introduction to the Poet Tree Club
- Including Files
- require
- require_once
- Constants
- Error Reporting
- Displaying Errors
- Including a Secure Configuration File
- Welcome to the Server-side
- PHP Conditionals
- if / if - else / if - elseif - else
- Simple if Condition
- if-else Condition
- if-elseif-else statement
- False Equivalents: Falsy Values
- Testing for Variable Existence
- Exercise: Checking for Variable Existence
- switch/case
- Ternary Operator
- Null Coalescing Operator
- if / if - else / if - elseif - else
- Arithmetic Operators and Loops
- Arithmetic Operators
- The Modulus Operator
- Loops
- while
- do...while
- for
- break and continue
- Arrays
- Indexed Arrays
- Initializing Arrays
- Appending to an Array
- Reading from Arrays
- Looping through Arrays
- Associative Arrays
- Initializing Associative Arrays
- Reading from Associative Arrays
- Looping through Associative Arrays
- Superglobal Arrays
- Multi-dimensional Arrays
- Reading from Two-dimensional Arrays
- Looping through Two-dimensional Arrays
- Two-dimensional Associative Arrays
- Non-tabular Multi-dimensional Arrays
- Array Manipulation Functions
- in_array() Function
- Indexed Arrays
- Working with Databases
- Objects
- Attributes / Properties
- Behaviors / Methods
- Classes vs Objects
- Connecting to a Database with PDO
- Introducing the Poetree Database
- phpMyAdmin
- Querying Records with PHP
- Queries Returning Multiple Rows
- Creating the Poems Listings
- Adding Pagination
- Sorting
- Filtering
- Exception Handling
- Uncaught Exceptions
- Throwing Your Own Exceptions
- Catching Exceptions
- Getting Information about Exceptions
- PDOExceptions
- When Queries Fail to Execute
- PHP and HTML Forms
- HTML Forms
- How HTML Forms Work
- Form Submissions
- Sanitizing Form Data
- htmlspecialchars()
- htmlentities()
- filter_var()
- filter_input()
- Validating Form Data
- Was the Field Filled In?
- Is the Entered Value an Integer?
- Is it an Email?
- Is it a Valid Password and Do the Passwords Match?
- Do the Combined Values Create a Valid Date?
- Did the User Check the Box?
- HTML Forms
- Sending Email with PHP
- mail()
- Shortcomings of mail()
- Setting Up PHPMailer
- Get and Install the Latest Version of PHPMailer
- Mail Server
- Including a Mail Configuration File
- Sending Email with PHPMailer
- PHPMailer Methods and Properties
- Creating a Contact Form
- mail()
- Authentication with PHP and SQL
- The Registration Process
- Passwords and Pass Phrases
- Registration with Tokens
- Creating a Registration Form
- Sessions and Session Variables
- Cookies
- Logging In and Out
- $_REQUEST Variables
- Resetting the Pass Phrase
- LAB: Inserting, Updating, and Deleting Poems
- Submitting a New Poem
- Editing an Existing Poem
- Deleting a Poem
- Uploading Files
- Uploading Images via an HTML Form
- Resizing Images
- Uploading a Profile Picture
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this PHP class:
- HTML
Experience in the following would be useful for this PHP class:
- CSS
- JavaScript
- Some SQL
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors
Self-Paced Course
- On Demand 24/7
- Readings
- Presentations
- Exercises
- Quizzes
- Full Year of Access
- Learn more