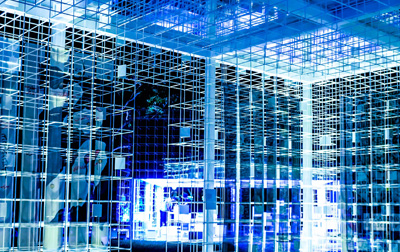
PHP and MySQL Training (PHP501)
This course provides a comprehensive introduction to developing dynamic, data-driven web applications using PHP and MySQL. Participants will gain foundational skills in both relational database design and server-side scripting, learning how to integrate PHP with MySQL to create interactive and robust web solutions. The course covers everything from basic SQL queries to advanced PHP functionalities, equipping learners with the tools needed to build real-world applications.
The course begins with Relational Database Basics, where you'll learn about the fundamentals of SQL, relational databases, and key concepts such as tables, rows, columns, primary and foreign keys, and popular database management systems. This module establishes the foundation necessary to understand how data is stored and managed within relational databases.
In Simple SELECTs, you will explore the basics of querying data with SQL. Topics include selecting columns, sorting records, using the WHERE clause for filtering, and working with logical operators. This section is designed to provide a strong grasp of how to retrieve and manipulate data within a MySQL database.
Advanced SELECTs delves deeper into SQL with calculated fields, aggregate functions, and grouping techniques. You will learn to manipulate data using built-in math, string, and date functions, and how to extract distinct records. This module enhances your ability to perform more complex data operations and analysis.
The Subqueries, Joins, and Unions module covers advanced data retrieval techniques. You will learn to work with subqueries, joins, and unions, understanding how to combine data from multiple tables to produce more comprehensive query results.
Next, the course transitions to PHP Basics, introducing you to server-side scripting. You’ll learn how PHP interacts with HTML, explore variable handling, write basic scripts, and understand the significance of including files. This module lays the groundwork for dynamic content generation and server-side logic.
In PHP Conditionals, you will learn how to control the flow of your application using if statements, switch/case, and ternary operators. Understanding conditionals is crucial for decision-making in code, allowing for more responsive and interactive applications.
Arithmetic Operators and Loops will guide you through using arithmetic operations and implementing loops in PHP. You will understand how to iterate through data sets and control repetitive tasks, enhancing the efficiency of your code.
The Arrays module introduces indexed, associative, and multidimensional arrays, along with key array manipulation functions. This section will help you understand how to store and access complex data structures efficiently.
In Working with Databases, you’ll learn how to connect PHP with MySQL using PDO, manage queries, and handle data effectively. Topics include pagination, sorting, and filtering data to ensure that your applications can interact seamlessly with a MySQL database.
Exception Handling focuses on managing errors in PHP. You’ll learn how to catch and handle exceptions, log errors, and ensure your applications are resilient to unexpected issues, providing a smoother user experience.
The PHP and HTML Forms module covers how to process user input through forms, including sanitizing and validating data to ensure it is safe and reliable. This section is critical for building interactive and secure web applications.
In Sending Email with PHP, you’ll explore techniques for sending emails using PHP's mail()
function and PHPMailer, an advanced library for managing email communication within web applications.
Authentication with PHP and SQL covers user registration, session management, and secure login/logout processes. You will learn how to authenticate users and manage cookies to keep your applications secure.
The course also includes a comprehensive lab, where you will apply your PHP and MySQL skills to build a functional web application that allows users to manage content dynamically.
Uploading Files will guide you through handling file uploads securely, including image resizing and managing user profile pictures. This module ensures you can safely handle and process file data within your applications.
Finally, the Admin Site module demonstrates how to create a backend interface for managing content and users, including permissions, approvals, and other administrative functions.
By the end of this course, you will have a solid foundation in PHP and MySQL, empowering you to build dynamic, data-driven web applications with robust server-side logic and database integration.
- Learn how PHP works.
- Learn the basic syntax of PHP.
- Learn to create dynamic interactive pages with PHP.
- Learn to manipulate files with PHP.
- Learn to work with arrays in PHP.
- Learn to validate forms with PHP.
- Learn to write functions in PHP.
- Learn to manipulate and manage database data with PHP.
- Learn to authenticate users with PHP.
- Learn to manage sessions with PHP.
- Learn to work with the MDB2 package.
- Learn advanced form validation with regular expressions.
- Learn to send email with PHP.
- Understand how MySQL works.
- Learn to use SQL to output reports with MySQL.
- Learn to modify MySQL data with SQL.
- Relational Database Basics
- Brief History of SQL
- Relational Databases
- Tables
- Rows
- Columns
- Relationships
- Datatypes
- Primary Keys
- Foreign Keys
- Relational Database Management System
- Popular Databases
- Commercial Databases
- Popular Open Source Databases
- SQL Statements
- Database Manipulation Language (DML)
- Database Definition Language (DDL)
- Database Control Language (DCL)
- Simple SELECTs
- Introduction to the Northwind Database
- Some Basics
- Comments
- Whitespace and Semi-colons
- Case Sensitivity
- SELECTing All Columns in All Rows
- SELECTing Specific Columns
- Sorting Records
- Sorting by a Single Column
- Sorting By Multiple Columns
- Ascending and Descending Sorts
- The WHERE Clause and Logical Operator Symbols
- Checking for Equality
- Checking for Inequality
- Checking for Greater or Less Than
- Checking for NULL
- WHERE and ORDER BY
- Checking Multiple Conditions with Boolean Operators
- AND
- OR
- Order of Evaluation
- The WHERE Clause and Logical Operator Keywords
- The BETWEEN Operator
- The IN Operator
- The LIKE Operator
- The NOT Operator
- Advanced SELECTs
- Calculated Fields
- Concatenation
- Mathematical Calculations
- Aliases
- Aggregate Functions and Grouping
- Aggregate Functions
- Grouping Data
- Selecting Distinct Records
- Built-in Data Manipulation Functions
- Common Math Functions
- Common String Functions
- Common Date Functions
- Calculated Fields
- Subqueries, Joins and Unions
- Subqueries
- Joins
- Table Aliases
- Multi-table Joins
- Outer Joins
- Unions
- UNION ALL
- UNION Rules
- PHP Basics
- Welcome to the Server-side
- Adding PHP Files to VS Code Workspace
- Google Chrome DevTools: Network Tab
- Review of DevTools Network Tab
- How PHP Works
- PHP Functions
- php.net
- Using php.net
- Variables
- Preparing to Write Your First PHP Code - Part 1
- Preparing to Write Your First PHP Code - Part 2
- Preparing to Write Your First PHP Code - Part 3
- First PHP Script
- Variable Scope
- Single Quotes vs. Double Quotes
- Concatenation
- Passing Variables on the URL
- User-defined Functions (UDFs)
- Local and Global Variables
- Passing Arguments by Value vs. by Reference
- Introduction to the Poet Tree Club
- Including Files
- Using Header and Footer Includes
- Constants
- Error Reporting
- Displaying Errors
- Including a Secure Configuration File
- Comments
- PHP Statements and Whitespace
- PHP Conditionals
- if / if - else / if - elseif - else
- Introduction to Conditionals
- False Equivalents: Falsy Values
- Testing for Variable Existence
- switch/case
- Ternary Operator
- The Ternary Operator
- Null Coalescing Operator
- Arithmetic Operators and Loops
- Arithmetic Operators
- The Modulus Operator
- Loops
- Modulus and Loops
- Arrays
- Indexed Arrays
- Working with Indexed Arrays
- Associative Arrays
- Superglobal Arrays
- Multi-dimensional Arrays
- Array Manipulation Functions
in_array()
Function
- Working with Databases
- Objects
- Attributes / Properties
- Behaviors / Methods
- Classes vs. Objects
- Connecting to a Database with PDO
- Introducing the Poetree Database
- phpMyAdmin
- Querying Records with PHP
- Queries Returning Multiple Rows
- Adding Pagination
- Sorting
- Anticipating Foul Play
- Filtering
- Exception Handling
- Uncaught Exceptions
- Throwing Your Own Exceptions
- Catching Exceptions
- Exceptions
- Division Form
- PDOExceptions
- Logging Errors
- The
dbConnect()
Function - When Queries Fail to Execute
- Preparing for the Catching Errors Exercise
- Catching Errors in the PHP Poetry Website
- PHP and HTML Forms
- Form Submissions
- Sanitizing Form Data
- Validating Form Data
- Processing Form Input: Trimming
- Processing Form Input: Filtering
- Processing Form Input: Validating Fields
- Processing Form Input: Submission Response
- Processing Form Input: Remembering Form Entries
- Sending Email with PHP
mail()
- Setting Up PHPMailer
- Mail Server
- Including a Mail Configuration File
- Sending Email with PHPMailer
- PHPMailer Methods and Properties
- Creating a Contact Form
- Authentication with PHP and SQL
- The Registration Process
- Passwords and Pass Phrases
- Registration with Tokens
- Creating a Registration Form
- Sessions
- Cookies
- Logging In and Out
$_REQUEST
Variables- Resetting the Pass Phrase Introduction
- LAB: Inserting, Updating, and Deleting Poems
- Submitting a New Poem
- Showing All User’s Poems in on the Poems Page
- Editing an Existing Poem
- Deleting a Poem
- Uploading Files
- Enabling File Info Functions on Windows
- Uploading Images via an HTML Form
- Resizing Images
- Uploading a Profile Picture
- Uploading Files
- Admin Site
- Adding the Admin Pages
- Creating the
isAdmin()
Function - Completing the Admin Home Page
- Completing the Admin Poems Page
- Approving, Editing, and Deleting Poems
- Completing the Admin Users Page
- Make the My Account Page Spoofable
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this PHP class:
- HTML
Experience in the following would be useful for this PHP class:
- CSS
- Basic Programming
- XML
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors