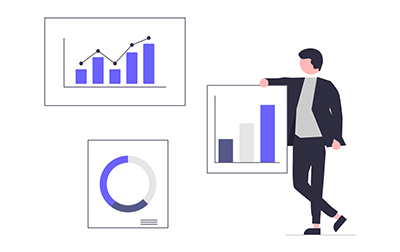
Introduction to R Programming (RPR101)
Course Length: 3 days
Delivery Methods:
Available as private class only
Course Overview
In this R training course, students learn to program in the R language. Whether you are learning R because you plan to use it for data science, data analytics, machine learning, statistical computing, this R course provides you with the prerequisite R programming knowledge to get you started.
Course Benefits
- Learn to create and use functions and packages.
- Learn to work with math in R.
- Learn to work with character data in R.
- Learn to work with dates and times in R.
- Learn to create vectors and lists.
- Learn to create conditional code and work with loops.
- Learn to handle dates and times.
- Learn to work with arrays and matrices.
- Learn to work with data frames.
- Learn to plot data with
matplot
.
Course Outline
- R Programming Basics
- Using R Studio
- Running R Statements
- Running an R File
- Exercise: Hello, World!
- Literals
- Exercise: Exploring Types
- Variables
- Exercise: A Simple R Script
- Constants and Deleting Variables
print()
Function- Using
paste()
andpaste0()
withprint()
- C-style Printing with
sprint()
- Collecting User Input
- Exercise: Hello, You!
- Functions and Packages
- Defining Functions
- Variables as Vectors
- Using
typeof()
andclass()
- Function Parameters
- Exercise: A Function with Parameters
- Returning Values
- Exercise: Parameters with Default Values
- Packages
- Math
- Arithmetic Operators
- Exercise: Floor and Modulus
- Assignment Operators
- Precedence of Operations
- Built-in Math Functions
- Using Random Numbers
- Exercise: How Many Pizzas Do We Need?
- Exercise: Dice Rolling
- Character
- Quotation Marks and Special Characters
- String Split
- Exercise: Splitting Strings
- Substring and Slicing
- Exercise: Substring
- Paste (Concatenate) Strings
- Exercise: Repetition
- Combining Concatenation and Repetition
- Exercise: Combining Concatenation and Repetition
- Vectors and Lists
- Definitions
- Atomic Vectors
- Lists (Non-atomic vectors)
- Sequences and Random
- Ranges
- Indexing using
[
and[[
- Exercise: Simple Rock, Paper, Scissors Game
$
operator- Exercise: Slicing Sequences
- Flow Control
- Conditional Statements
- Compound Conditions
- The
is
andis not
Operators all()
andany()
and the Ternary Operator- Loops in R
- Exercise: All True and Any True
- break and next
- Exception Handling
- Exception Basics
try()
tryCatch()
- Dates and Times
date()
Sys.Date()
Sys.time()
- Dates as Strings
- Time and Formatted Strings
- Exercise: What Color Pants Should I Wear?
- Date and Time arithmetic
- Exercise: Report on Departure Times
- Matrices and Arrays
- What is a Matrix?
- Creating a Matrix
rbind()
andcbind()
matrix()
- Performing Arithmetic on Matrices
- Retrieving Rows and Columns
- Exercise: Working with an Array
- What is an Array?
- Creating an Array
- Naming Rows and Columns
- Exercise: Working with an Array
- Data Frames
- What is a Data Frame?
- Creating a Data Frame using
data.frame()
- Creating a Data Frame from a CSV
- Exploring a Data Frame using
str()
andsummary()
- Exercise: Practice Exploring a Data Frame
- Changing Values
- Retrieving Rows and Columns
- Combining Row and Column Selection
- Adding a Column to the Data Frame
- Add a Row to the Data Frame with
rbind()
- Plotting with
matplot
- Exercise: Plotting a Data Frame
- Other Kinds of Plots
Class Materials
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors