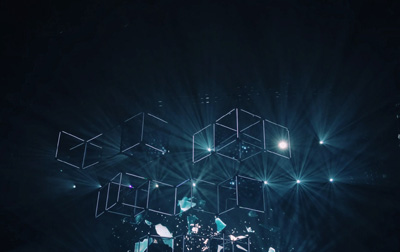
Test-Driven Development (TDD) Using React.js and ES6 (TDD101)
In this 5-day Test-Driven Development (TDD) Using React.js and ES6 training class, students will gain hands-on experience with some of the most current tools, techniques, and technologies in front-end web development.
This Test-driven Development (TDD) Using React and ES6 training class is a complete introduction to modern front-end development. It covers the syntax, conventions, and best practices of modern JavaScript development while also teaching React. In the process, students learn about TDD and, specifically, how to test React and Redux applications using the Jest testing framework as they build a complete React/Redux application.
This course is ideal for experienced software developers who are new to JavaScript and TDD or for JavaScript developers who may be new to ES6+ and TDD.
- Learn how to install, configure and use modern web tooling.
- Understand test-driven development.
- Learn to create test suites using Jasmine.
- Write ES6 code and compile it using Babel.
- Understand what React.js is and what problem it solves.
- Explore the basic architecture of a React.js application.
- Gain a deep knowledge of React.js components and JSX.
- Build a working application that uses React.js components.
- Use Redux for maintaining state in a React.js application.
- Learn React.js best practices.
- React Quickstart with Create-React-App
- Development Ecosystem
- Code Editors and IDEs
- Lab 1: Installing and Configuring WebStorm IDE
- Node.js
- EventEmitter
- Node Streams
- Node Modules
- Lab 2 - Getting Started with Node.js
- Git
- What is Version Control
- History of Git
- What is Git?
- 3 States of Git
- Git Workflow
- Lab 3 - Version Control With Git
- Command Prompt
- Know Your Shell
- Reproducible Builds
- Why Automate Your Build?
- Build Requirements
- npm
- Lab 4 – Initialize npm
- node_modules
- package.json
- npm install
- Lab 5 – Using npm as a Build Tool
- Lab 6 - Managing External Dependencies
- Static Code Analysis
- Lint tools
- Configuring ESLint
- ESLint: What Can Be Configured?
- ESLint Rules
- Lab 7 - Automate Linting
- Lab 8 - Configure a Local Web Server
- Browser Development Tools
- Test-Driven Development
- Goal of TDD
- The TDD Cycle
- Red, Green, Refactor
- Assertions
- JavaScript Testing Frameworks
- JS Exception Handling
- Jasmine Overview
- How Jasmine Works
- Test Suites
- Specs
- Expectations
- Matchers
- TDD vs. BDD
- Lab 9 – Get Started with Jasmine
- Lab 10 - TDD Practice
- Automated Cross-browser Testing
- Karma
- Lab 11 - In-browser Testing with Karma
- Modularity
- Why is Modularity Important?
- CommonJS
- RequireJS
- ES6 Modules
- Front-end Modules
- Manage Modules Manually
- Front End Package Management with npm
- Building and Refactoring
- Building the dist directory
- webpack
- Lab 12: Deploying with Webpack
- Lab 13 README Update and Refactoring
- ES2015 (ES6)
- Variable Scoping with const and let
- let vs. var
- Block-scoped Functions
- Arrow Functions
- Default Parameter Handling
- Rest Parameter
- Spread Operator
- Template Literals
- Enhanced Object Properties
- Array Matching
- Object Matching
- Symbol Primitive
- User-defined Iterators
- For-Of Operator
- Creating and Consuming Generator Functions
- Class Definition
- Class Declaration
- Class Expressions
- Class Inheritance
- Advanced JavaScript Topics
- “use strict”
- Understanding this
- 4 Rules of this
- What is this?
- Implicit Binding
- Explicit Binding
- new Binding
- window Binding
- Array.map()
- Promises
- Promises vs. Event Listeners
- Why use Promises?
- Demo: Callback vs. Promise
- Using Promises
- Babel
- Lab 14: Transpiling with Babel
- Lab 15: Converting to ES6
- The Document Object Model
- What is the DOM?
- Understanding Nodes
- EventTarget
- DOM Events
- Other Events
- Element
- Manipulating HTML with the DOM
- Manipulating HTML with JQuery
- Manipulating HTML with React
- Introduction to React.js
- What is React.js
- Imperative API vs. Declarative API
- Imperative vs. Declarative Screen updates
- One-way Data Flow
- Virtual DOM
- Virtual DOM vs. HTML DOM
- State Machines
- Lab 16, Part 1: Hello, React!
- Understanding Components
- React.render()
- ReactDOM
- React Development Process
- Props vs. State
- Setting Initial State
- super()
- Lab 16, Parts 2-3: Your first Component
- JSX
- What is JSX?
- Using JSX
- JSX is not Exactly HTML
- Using React with JSX
- Using React without JSX
- Expressions in JSX
- Precompiled JSX
- Lab 17 - HTML to JSX
- React Components
- Creating Components
- Pure Functions
- Benefits of Pure Functions
- F.I.R.S.T
- Single Responsibility
- Communication Between Components
- Props
- Ref Callback
- Lab 18: Passing Props
- Styles in React
- Style Components
- Lab 19: Style in React
- Forms
- Forms Have State
- Form Events
- Controlled Components
- Uncontrolled Components
- Lab 20: Controlling the Form
- Stateless Functional Components
- Lab 21: Refactoring the App
- Component Life-Cycle Events
- Life-Cycle Methods
- Mount/Unmount
- Mount/Unmount Life-Cycle Methods
- Data Life-Cycle Methods
- Component Life Cycle
- Events
- Lab 22: Life Cycle and Events
- Composition
- Reusable Components
- Presentational Components
- Container Components
- PropTypes
- Lab 23: PropTypes
- Testing React Components
- What to Test in a React Component
- Jest
- Mocking
- Snapshot Testing
- TestUtils
- Enzyme
- Shallow Rendering
- Lab 24: Testing React Components
- Lab 24.5: Testing with Jest and Enzyme
- Lab 25: Multiple Components
- React Router
- Lab 26: React Router
- Lab 27: React Router, Part 2
- Flux and Redux
- Flux
- Flux Flow
- Flux Action
- Flux Dispatcher
- Flux Stores
- EventEmitter
- Redux
- Stores & Immutable State Tree
- Redux Actions
- Reducers
- Things You Should Never Do in a Reducer
- Reducer Composition
- Redux Store
- Redux Pros and Cons
- Lab 28: Redux Thermometer
- Lab 29: Implementing Redux
- React AJAX Best Practices
- Redux with Ajax
- What is Redux Middleware?
- What is Middleware Good For?
- Thunk
- Redux Saga
- Using Sagas
- Lab 30: SwimCalc App
- Lab 31: Redux Middleware
- create-react-app
- Lab 32: create-react-app and enzyme
- Relay and GraphQL
- Advanced Topics
- Server-side React
- Using React with Other Libraries
- Performance Optimization
- Development vs. Production
- Perf Object
- Perf Object Methods
- Optimization Techniques
- Using pre-built Components
- Further Study
Each student will receive a comprehensive set of materials, including course notes and all the class examples.
Experience in the following is required for this React class:
- HTML and CSS
- Some JavaScript experience
- Familiarity with intermediate-to-advanced JavaScript programming topics, including objects, functions, closures, callbacks, prototypes and object-oriented JavaScript
Courses that can help you meet these prerequisites:
Live Private Class
- Private Class for your Team
- Live training
- Online or On-location
- Customizable
- Expert Instructors